What is the code for adding a tab to an HTML page that displays real-time cryptocurrency data?

I would like to know how to add a tab to an HTML page that can display real-time cryptocurrency data. Can you provide me with the code or steps to achieve this? I want the tab to show the latest prices and other relevant information for different cryptocurrencies.
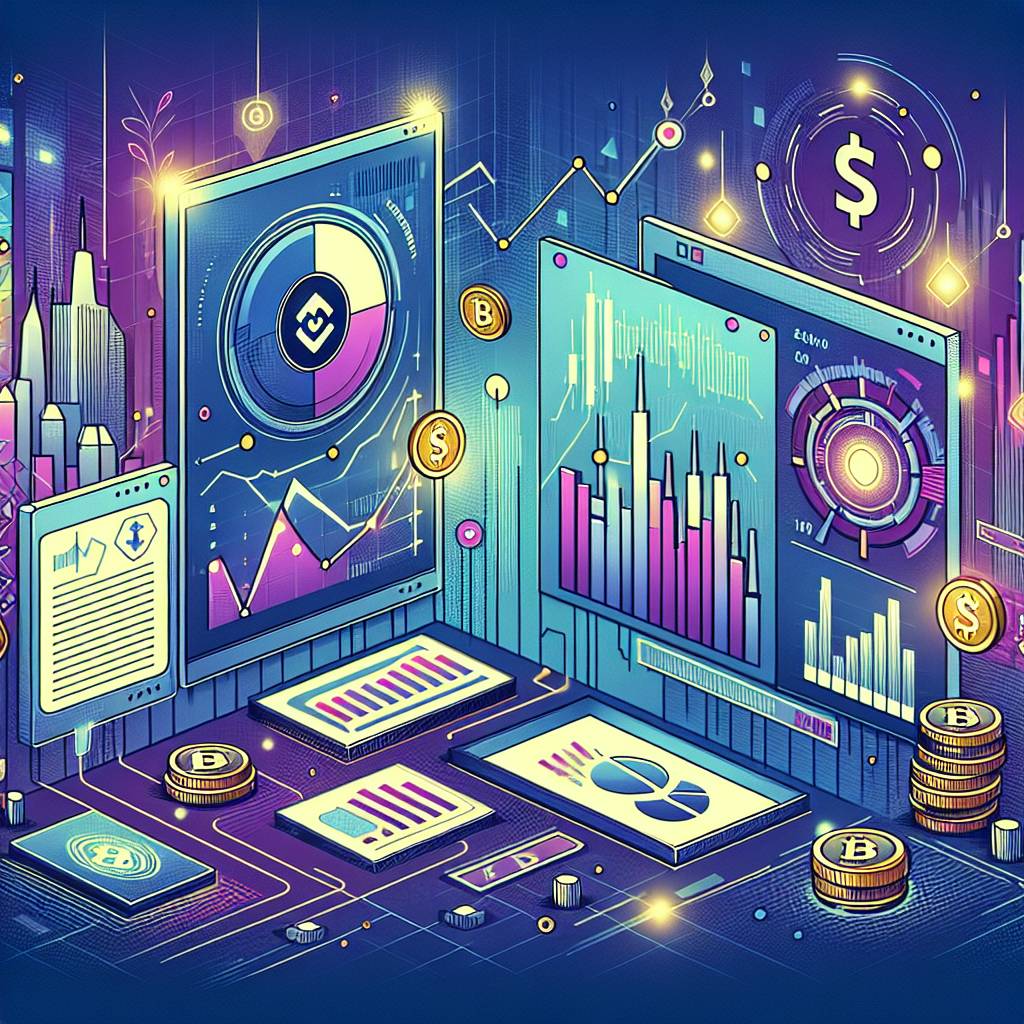
5 answers
- Sure! To add a tab to an HTML page that displays real-time cryptocurrency data, you can use HTML, CSS, and JavaScript. Here's a simple example: HTML: <div class="tab"> <button class="tablinks" onclick="openTab(event, 'CryptoData')">Crypto Data</button> </div> <div id="CryptoData" class="tabcontent"> <h3>Real-time Cryptocurrency Data</h3> <p>This is where you can display the real-time cryptocurrency data.</p> </div> CSS: .tab { overflow: hidden; } .tab button { background-color: #f1f1f1; border: none; outline: none; cursor: pointer; padding: 10px 20px; transition: 0.3s; font-size: 16px; } .tab button:hover { background-color: #ddd; } .tabcontent { display: none; padding: 20px; border: 1px solid #ccc; } JavaScript: function openTab(evt, tabName) { var i, tabcontent, tablinks; tabcontent = document.getElementsByClassName("tabcontent"); for (i = 0; i < tabcontent.length; i++) { tabcontent[i].style.display = "none"; } tablinks = document.getElementsByClassName("tablinks"); for (i = 0; i < tablinks.length; i++) { tablinks[i].className = tablinks[i].className.replace(" active", ""); } document.getElementById(tabName).style.display = "block"; evt.currentTarget.className += " active"; } You can customize the code to fetch real-time cryptocurrency data from an API and display it in the 'CryptoData' tab. Remember to include the necessary CSS styles to make the tab look visually appealing.
Nov 26, 2021 · 3 years ago
- Adding a tab to an HTML page that displays real-time cryptocurrency data requires some coding skills. You can achieve this by using HTML, CSS, and JavaScript. Here's a basic example: HTML: <div class="tab"> <button class="tablinks" onclick="openTab(event, 'CryptoData')">Crypto Data</button> </div> <div id="CryptoData" class="tabcontent"> <h3>Real-time Cryptocurrency Data</h3> <p>This is where you can display the real-time cryptocurrency data.</p> </div> CSS: .tab { overflow: hidden; } .tab button { background-color: #f1f1f1; border: none; outline: none; cursor: pointer; padding: 10px 20px; transition: 0.3s; font-size: 16px; } .tab button:hover { background-color: #ddd; } .tabcontent { display: none; padding: 20px; border: 1px solid #ccc; } JavaScript: function openTab(evt, tabName) { var i, tabcontent, tablinks; tabcontent = document.getElementsByClassName("tabcontent"); for (i = 0; i < tabcontent.length; i++) { tabcontent[i].style.display = "none"; } tablinks = document.getElementsByClassName("tablinks"); for (i = 0; i < tablinks.length; i++) { tablinks[i].className = tablinks[i].className.replace(" active", ""); } document.getElementById(tabName).style.display = "block"; evt.currentTarget.className += " active"; } You can modify the code to fetch real-time cryptocurrency data from an API and display it in the 'CryptoData' tab. Make sure to style the tab and its content using CSS to match your website's design.
Nov 26, 2021 · 3 years ago
- No problem! To add a tab to an HTML page that displays real-time cryptocurrency data, you can use HTML, CSS, and JavaScript. Here's an example code snippet: HTML: <div class="tab"> <button class="tablinks" onclick="openTab(event, 'CryptoData')">Crypto Data</button> </div> <div id="CryptoData" class="tabcontent"> <h3>Real-time Cryptocurrency Data</h3> <p>This is where you can display the real-time cryptocurrency data.</p> </div> CSS: .tab { overflow: hidden; } .tab button { background-color: #f1f1f1; border: none; outline: none; cursor: pointer; padding: 10px 20px; transition: 0.3s; font-size: 16px; } .tab button:hover { background-color: #ddd; } .tabcontent { display: none; padding: 20px; border: 1px solid #ccc; } JavaScript: function openTab(evt, tabName) { var i, tabcontent, tablinks; tabcontent = document.getElementsByClassName("tabcontent"); for (i = 0; i < tabcontent.length; i++) { tabcontent[i].style.display = "none"; } tablinks = document.getElementsByClassName("tablinks"); for (i = 0; i < tablinks.length; i++) { tablinks[i].className = tablinks[i].className.replace(" active", ""); } document.getElementById(tabName).style.display = "block"; evt.currentTarget.className += " active"; } Feel free to customize the code to fit your needs. You can fetch real-time cryptocurrency data from various APIs and display it in the 'CryptoData' tab. Remember to style the tab using CSS to make it visually appealing.
Nov 26, 2021 · 3 years ago
- Well, if you want to add a tab to an HTML page that displays real-time cryptocurrency data, you'll need to use HTML, CSS, and JavaScript. Here's a code snippet that can help you achieve that: HTML: <div class="tab"> <button class="tablinks" onclick="openTab(event, 'CryptoData')">Crypto Data</button> </div> <div id="CryptoData" class="tabcontent"> <h3>Real-time Cryptocurrency Data</h3> <p>This is where you can display the real-time cryptocurrency data.</p> </div> CSS: .tab { overflow: hidden; } .tab button { background-color: #f1f1f1; border: none; outline: none; cursor: pointer; padding: 10px 20px; transition: 0.3s; font-size: 16px; } .tab button:hover { background-color: #ddd; } .tabcontent { display: none; padding: 20px; border: 1px solid #ccc; } JavaScript: function openTab(evt, tabName) { var i, tabcontent, tablinks; tabcontent = document.getElementsByClassName("tabcontent"); for (i = 0; i < tabcontent.length; i++) { tabcontent[i].style.display = "none"; } tablinks = document.getElementsByClassName("tablinks"); for (i = 0; i < tablinks.length; i++) { tablinks[i].className = tablinks[i].className.replace(" active", ""); } document.getElementById(tabName).style.display = "block"; evt.currentTarget.className += " active"; } You can customize the code to fetch real-time cryptocurrency data from an API and display it in the 'CryptoData' tab. Don't forget to style the tab using CSS to make it look good.
Nov 26, 2021 · 3 years ago
- BYDFi is a great platform for trading cryptocurrencies, and they provide an easy way to add a tab to an HTML page that displays real-time cryptocurrency data. Here's a simple code snippet: HTML: <div class="tab"> <button class="tablinks" onclick="openTab(event, 'CryptoData')">Crypto Data</button> </div> <div id="CryptoData" class="tabcontent"> <h3>Real-time Cryptocurrency Data</h3> <p>This is where you can display the real-time cryptocurrency data.</p> </div> CSS: .tab { overflow: hidden; } .tab button { background-color: #f1f1f1; border: none; outline: none; cursor: pointer; padding: 10px 20px; transition: 0.3s; font-size: 16px; } .tab button:hover { background-color: #ddd; } .tabcontent { display: none; padding: 20px; border: 1px solid #ccc; } JavaScript: function openTab(evt, tabName) { var i, tabcontent, tablinks; tabcontent = document.getElementsByClassName("tabcontent"); for (i = 0; i < tabcontent.length; i++) { tabcontent[i].style.display = "none"; } tablinks = document.getElementsByClassName("tablinks"); for (i = 0; i < tablinks.length; i++) { tablinks[i].className = tablinks[i].className.replace(" active", ""); } document.getElementById(tabName).style.display = "block"; evt.currentTarget.className += " active"; } You can modify the code to fetch real-time cryptocurrency data from BYDFi's API and display it in the 'CryptoData' tab. Make sure to style the tab and its content using CSS to match your website's design.
Nov 26, 2021 · 3 years ago
Related Tags
Hot Questions
- 87
How does cryptocurrency affect my tax return?
- 85
What are the best digital currencies to invest in right now?
- 66
What is the future of blockchain technology?
- 45
What are the best practices for reporting cryptocurrency on my taxes?
- 33
What are the tax implications of using cryptocurrency?
- 31
How can I minimize my tax liability when dealing with cryptocurrencies?
- 18
What are the advantages of using cryptocurrency for online transactions?
- 15
How can I buy Bitcoin with a credit card?