What are the best ways to add an element to an array in a PHP script for cryptocurrency transactions?

I'm working on a PHP script for cryptocurrency transactions, and I need to add an element to an array. What are the best ways to achieve this in PHP? I want to make sure that the added element is properly formatted and can be easily accessed later in the script. Can you provide some guidance on how to accomplish this?
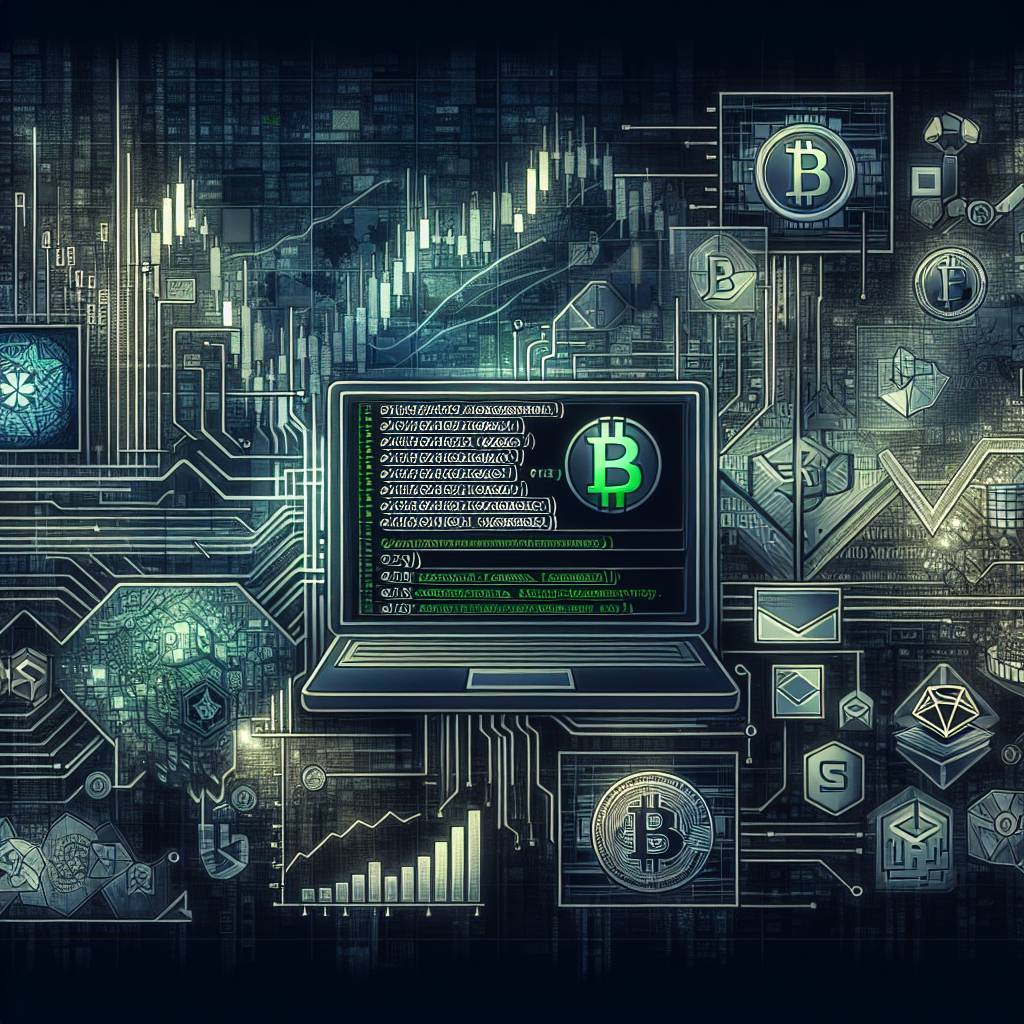
6 answers
- One of the best ways to add an element to an array in a PHP script for cryptocurrency transactions is by using the array_push() function. This function allows you to add one or more elements to the end of an array. Here's an example: ``` $myArray = array('Bitcoin', 'Ethereum'); array_push($myArray, 'Ripple'); print_r($myArray); ``` This will output: ``` Array ( [0] => Bitcoin [1] => Ethereum [2] => Ripple ) ``` You can also use the shorthand notation `$myArray[] = 'Ripple';` to achieve the same result. Both methods are commonly used and should work well for your cryptocurrency transactions.
Dec 17, 2021 · 3 years ago
- To add an element to an array in a PHP script for cryptocurrency transactions, you can use the array_merge() function. This function allows you to merge two or more arrays together, including adding new elements. Here's an example: ``` $myArray = array('Bitcoin', 'Ethereum'); $newElement = array('Ripple'); $mergedArray = array_merge($myArray, $newElement); print_r($mergedArray); ``` This will output: ``` Array ( [0] => Bitcoin [1] => Ethereum [2] => Ripple ) ``` Using array_merge() gives you more flexibility if you need to add multiple elements or merge multiple arrays at once.
Dec 17, 2021 · 3 years ago
- Adding an element to an array in a PHP script for cryptocurrency transactions is a common task. One way to achieve this is by using the `[]` notation. Here's an example: ``` $myArray = ['Bitcoin', 'Ethereum']; $myArray[] = 'Ripple'; print_r($myArray); ``` This will output: ``` Array ( [0] => Bitcoin [1] => Ethereum [2] => Ripple ) ``` Using the `[]` notation is simple and concise, and it allows you to add elements to an array without the need for any additional functions or methods.
Dec 17, 2021 · 3 years ago
- When it comes to adding an element to an array in a PHP script for cryptocurrency transactions, one approach you can consider is using the `array_push()` function. This function allows you to add one or more elements to the end of an array. Here's an example: ``` $myArray = array('Bitcoin', 'Ethereum'); array_push($myArray, 'Ripple'); print_r($myArray); ``` This will output: ``` Array ( [0] => Bitcoin [1] => Ethereum [2] => Ripple ) ``` Using `array_push()` is a convenient way to add elements to an array, especially if you need to add multiple elements at once.
Dec 17, 2021 · 3 years ago
- To add an element to an array in a PHP script for cryptocurrency transactions, you can use the `array_push()` function. This function allows you to add one or more elements to the end of an array. Here's an example: ``` $myArray = array('Bitcoin', 'Ethereum'); array_push($myArray, 'Ripple'); print_r($myArray); ``` This will output: ``` Array ( [0] => Bitcoin [1] => Ethereum [2] => Ripple ) ``` Using `array_push()` is a straightforward way to add elements to an array in PHP, and it works well for cryptocurrency transactions.
Dec 17, 2021 · 3 years ago
- When you need to add an element to an array in a PHP script for cryptocurrency transactions, you can use the `array_push()` function. This function allows you to add one or more elements to the end of an array. Here's an example: ``` $myArray = array('Bitcoin', 'Ethereum'); array_push($myArray, 'Ripple'); print_r($myArray); ``` This will output: ``` Array ( [0] => Bitcoin [1] => Ethereum [2] => Ripple ) ``` Using `array_push()` is a reliable method for adding elements to an array in PHP, and it's commonly used in cryptocurrency transactions.
Dec 17, 2021 · 3 years ago
Related Tags
Hot Questions
- 97
Are there any special tax rules for crypto investors?
- 89
How does cryptocurrency affect my tax return?
- 82
What is the future of blockchain technology?
- 73
How can I buy Bitcoin with a credit card?
- 65
What are the tax implications of using cryptocurrency?
- 61
What are the best digital currencies to invest in right now?
- 37
What are the best practices for reporting cryptocurrency on my taxes?
- 30
How can I protect my digital assets from hackers?