What are some examples of Python code for accessing and analyzing data from the Etherscan API?

Can you provide some examples of Python code that can be used to access and analyze data from the Etherscan API? I'm interested in learning how to retrieve and process data related to cryptocurrencies using Python.
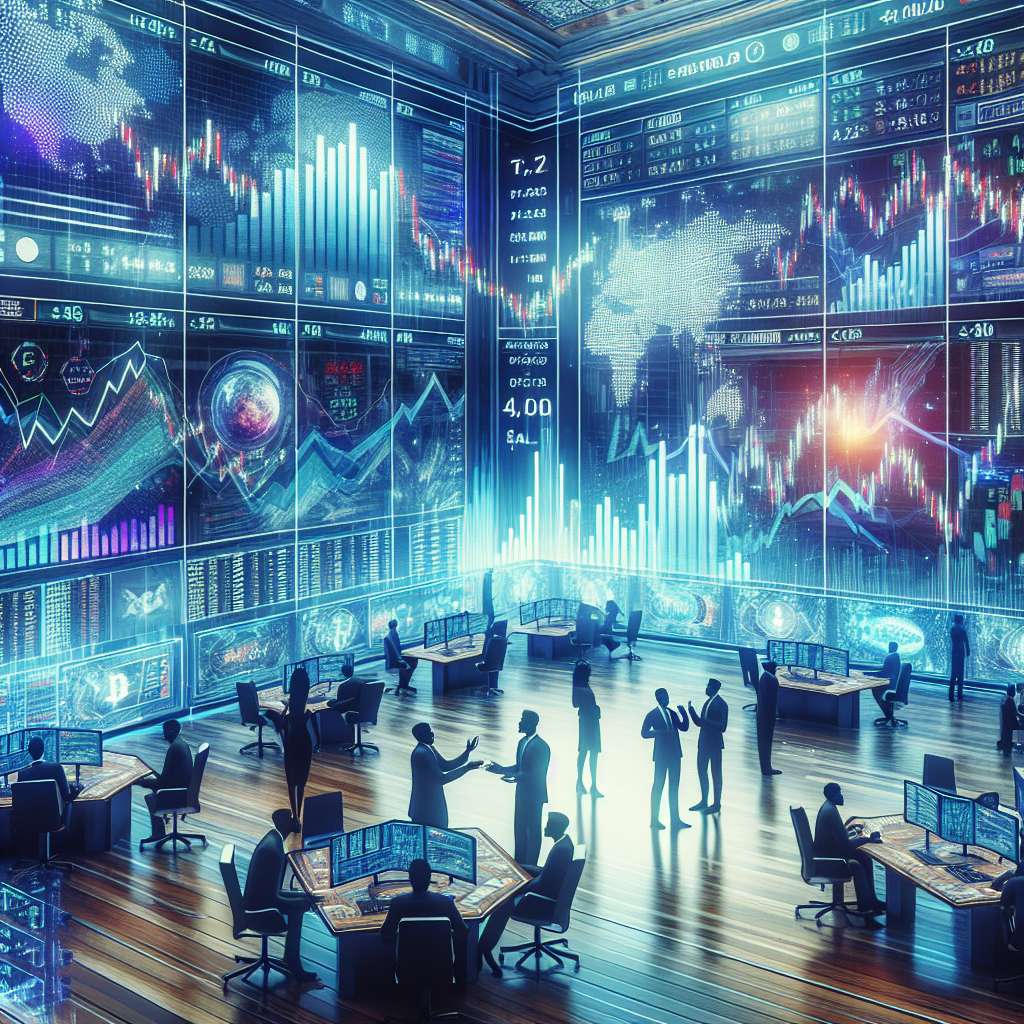
3 answers
- Sure! Here's an example of Python code that uses the Etherscan API to retrieve the balance of a specific Ethereum address: ```python import requests address = '0x123456789abcdef' api_key = 'your_api_key' url = f'https://api.etherscan.io/api?module=account&action=balance&address={address}&apikey={api_key}' response = requests.get(url) if response.status_code == 200: balance = int(response.json()['result']) / 10**18 print(f'The balance of {address} is {balance} ETH') else: print('Error retrieving balance') ```
Nov 29, 2021 · 3 years ago
- Here's another example of Python code that uses the Etherscan API to retrieve the transaction history of a specific Ethereum address: ```python import requests address = '0x123456789abcdef' api_key = 'your_api_key' url = f'https://api.etherscan.io/api?module=account&action=txlist&address={address}&apikey={api_key}' response = requests.get(url) if response.status_code == 200: transactions = response.json()['result'] for tx in transactions: print(f'Transaction hash: {tx['hash']}') print(f'From: {tx['from']}') print(f'To: {tx['to']}') print(f'Value: {int(tx['value']) / 10**18} ETH') print('---') else: print('Error retrieving transaction history') ```
Nov 29, 2021 · 3 years ago
- BYDFi provides a Python library called 'etherscan-python' that simplifies the process of accessing and analyzing data from the Etherscan API. You can install it using pip: ```bash pip install etherscan-python ``` Once installed, you can use it to retrieve data such as balances, transaction history, and token transfers. Here's an example: ```python from etherscan import Etherscan address = '0x123456789abcdef' api_key = 'your_api_key' eth = Etherscan(api_key) balance = eth.get_balance(address) print(f'The balance of {address} is {balance} ETH') transactions = eth.get_transaction_history(address) for tx in transactions: print(f'Transaction hash: {tx['hash']}') print(f'From: {tx['from']}') print(f'To: {tx['to']}') print(f'Value: {tx['value']} ETH') print('---') ```
Nov 29, 2021 · 3 years ago
Related Tags
Hot Questions
- 78
How can I buy Bitcoin with a credit card?
- 71
How does cryptocurrency affect my tax return?
- 69
What are the tax implications of using cryptocurrency?
- 60
What are the best digital currencies to invest in right now?
- 55
How can I minimize my tax liability when dealing with cryptocurrencies?
- 49
What is the future of blockchain technology?
- 45
How can I protect my digital assets from hackers?
- 30
Are there any special tax rules for crypto investors?