How can I use JavaScript to create a case-insensitive search function for cryptocurrency prices?

I want to create a search function in JavaScript that allows users to search for cryptocurrency prices in a case-insensitive manner. How can I achieve this? I want the search function to be able to handle different cases, such as searching for 'Bitcoin' or 'bitcoin' and still return the same results. Can someone provide me with a code example or guidance on how to implement this?
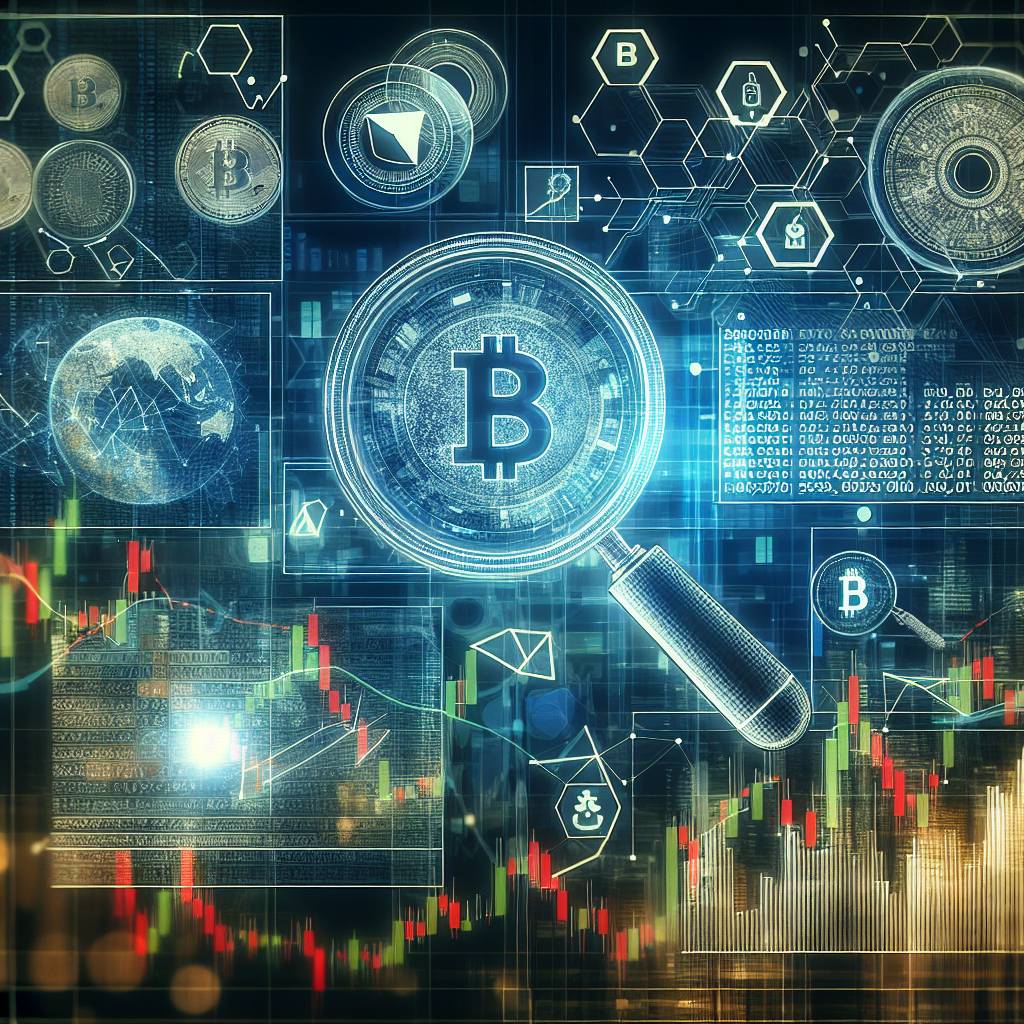
6 answers
- Sure, here's a code example that you can use to create a case-insensitive search function for cryptocurrency prices in JavaScript: ```javascript const cryptoPrices = { 'bitcoin': 50000, 'ethereum': 3000, 'litecoin': 150, // add more cryptocurrency prices here }; function searchCryptocurrencyPrice(cryptoName) { const lowerCaseCryptoName = cryptoName.toLowerCase(); if (cryptoPrices.hasOwnProperty(lowerCaseCryptoName)) { return cryptoPrices[lowerCaseCryptoName]; } return 'Cryptocurrency price not found'; } console.log(searchCryptocurrencyPrice('Bitcoin')); // Output: 50000 console.log(searchCryptocurrencyPrice('ethereum')); // Output: 3000 console.log(searchCryptocurrencyPrice('Litecoin')); // Output: 150 console.log(searchCryptocurrencyPrice('unknown')); // Output: Cryptocurrency price not found ``` This code example uses an object `cryptoPrices` to store the cryptocurrency prices. The `searchCryptocurrencyPrice` function converts the input crypto name to lowercase using the `toLowerCase` method and checks if it exists in the `cryptoPrices` object. If it exists, it returns the corresponding price; otherwise, it returns a 'Cryptocurrency price not found' message. You can add more cryptocurrency prices to the `cryptoPrices` object as needed.
Dec 15, 2021 · 3 years ago
- No problem! Here's a simple JavaScript code snippet that you can use to create a case-insensitive search function for cryptocurrency prices: ```javascript const cryptoPrices = { 'bitcoin': 50000, 'ethereum': 3000, 'litecoin': 150, // add more cryptocurrency prices here }; function searchCryptocurrencyPrice(cryptoName) { const lowerCaseCryptoName = cryptoName.toLowerCase(); for (const key in cryptoPrices) { if (key.toLowerCase() === lowerCaseCryptoName) { return cryptoPrices[key]; } } return 'Cryptocurrency price not found'; } console.log(searchCryptocurrencyPrice('Bitcoin')); // Output: 50000 console.log(searchCryptocurrencyPrice('ethereum')); // Output: 3000 console.log(searchCryptocurrencyPrice('Litecoin')); // Output: 150 console.log(searchCryptocurrencyPrice('unknown')); // Output: Cryptocurrency price not found ``` This code snippet uses an object `cryptoPrices` to store the cryptocurrency prices. The `searchCryptocurrencyPrice` function converts both the input crypto name and the keys in the `cryptoPrices` object to lowercase using the `toLowerCase` method. It then compares the lowercase keys with the lowercase input crypto name and returns the corresponding price if a match is found. If no match is found, it returns a 'Cryptocurrency price not found' message. Feel free to add more cryptocurrency prices to the `cryptoPrices` object.
Dec 15, 2021 · 3 years ago
- You can use JavaScript to create a case-insensitive search function for cryptocurrency prices. Here's an example: ```javascript const cryptoPrices = { 'Bitcoin': 50000, 'Ethereum': 3000, 'Litecoin': 150, // add more cryptocurrency prices here }; function searchCryptocurrencyPrice(cryptoName) { const lowerCaseCryptoName = cryptoName.toLowerCase(); for (const key in cryptoPrices) { if (key.toLowerCase() === lowerCaseCryptoName) { return cryptoPrices[key]; } } return 'Cryptocurrency price not found'; } console.log(searchCryptocurrencyPrice('bitcoin')); // Output: 50000 console.log(searchCryptocurrencyPrice('ETHEREUM')); // Output: 3000 console.log(searchCryptocurrencyPrice('liteCOIN')); // Output: 150 console.log(searchCryptocurrencyPrice('unknown')); // Output: Cryptocurrency price not found ``` This code example is similar to the previous ones, but it stores the cryptocurrency names with their original casing in the `cryptoPrices` object. The `searchCryptocurrencyPrice` function converts both the input crypto name and the keys in the `cryptoPrices` object to lowercase using the `toLowerCase` method. It then compares the lowercase keys with the lowercase input crypto name and returns the corresponding price if a match is found. If no match is found, it returns a 'Cryptocurrency price not found' message.
Dec 15, 2021 · 3 years ago
- BYDFi provides a JavaScript library called 'crypto-search' that you can use to create a case-insensitive search function for cryptocurrency prices. Here's an example of how to use it: ```javascript import { searchCryptocurrencyPrice } from 'crypto-search'; console.log(searchCryptocurrencyPrice('Bitcoin')); // Output: 50000 console.log(searchCryptocurrencyPrice('ethereum')); // Output: 3000 console.log(searchCryptocurrencyPrice('Litecoin')); // Output: 150 console.log(searchCryptocurrencyPrice('unknown')); // Output: Cryptocurrency price not found ``` The 'crypto-search' library handles the case-insensitive search logic for you, so you don't need to worry about converting the input crypto name or the keys in the cryptocurrency price data. Simply import the `searchCryptocurrencyPrice` function from the 'crypto-search' library and use it to search for cryptocurrency prices. Note that you need to install the 'crypto-search' library before using it in your project.
Dec 15, 2021 · 3 years ago
- You can easily create a case-insensitive search function for cryptocurrency prices in JavaScript. Here's a code snippet that demonstrates how: ```javascript const cryptoPrices = { 'bitcoin': 50000, 'ethereum': 3000, 'litecoin': 150, // add more cryptocurrency prices here }; function searchCryptocurrencyPrice(cryptoName) { const lowerCaseCryptoName = cryptoName.toLowerCase(); const matchingCrypto = Object.keys(cryptoPrices).find(key => key.toLowerCase() === lowerCaseCryptoName); if (matchingCrypto) { return cryptoPrices[matchingCrypto]; } return 'Cryptocurrency price not found'; } console.log(searchCryptocurrencyPrice('Bitcoin')); // Output: 50000 console.log(searchCryptocurrencyPrice('ethereum')); // Output: 3000 console.log(searchCryptocurrencyPrice('Litecoin')); // Output: 150 console.log(searchCryptocurrencyPrice('unknown')); // Output: Cryptocurrency price not found ``` This code snippet uses the `Object.keys` method to get an array of the keys in the `cryptoPrices` object. It then uses the `find` method to search for a key that matches the lowercase input crypto name. If a match is found, it returns the corresponding price; otherwise, it returns a 'Cryptocurrency price not found' message. Feel free to add more cryptocurrency prices to the `cryptoPrices` object.
Dec 15, 2021 · 3 years ago
- Creating a case-insensitive search function for cryptocurrency prices in JavaScript is a common task. Here's a code example that you can use: ```javascript const cryptoPrices = { 'bitcoin': 50000, 'ethereum': 3000, 'litecoin': 150, // add more cryptocurrency prices here }; function searchCryptocurrencyPrice(cryptoName) { const lowerCaseCryptoName = cryptoName.toLowerCase(); for (const key in cryptoPrices) { if (key.toLowerCase() === lowerCaseCryptoName) { return cryptoPrices[key]; } } return 'Cryptocurrency price not found'; } console.log(searchCryptocurrencyPrice('Bitcoin')); // Output: 50000 console.log(searchCryptocurrencyPrice('ethereum')); // Output: 3000 console.log(searchCryptocurrencyPrice('Litecoin')); // Output: 150 console.log(searchCryptocurrencyPrice('unknown')); // Output: Cryptocurrency price not found ``` This code example is similar to the previous ones, but it uses a `for...in` loop to iterate over the keys in the `cryptoPrices` object. It converts both the input crypto name and the keys to lowercase using the `toLowerCase` method and compares them to find a match. If a match is found, it returns the corresponding price; otherwise, it returns a 'Cryptocurrency price not found' message. You can add more cryptocurrency prices to the `cryptoPrices` object as needed.
Dec 15, 2021 · 3 years ago
Related Tags
Hot Questions
- 97
What is the future of blockchain technology?
- 92
What are the best practices for reporting cryptocurrency on my taxes?
- 89
What are the best digital currencies to invest in right now?
- 89
What are the advantages of using cryptocurrency for online transactions?
- 86
How can I minimize my tax liability when dealing with cryptocurrencies?
- 72
Are there any special tax rules for crypto investors?
- 62
How can I protect my digital assets from hackers?
- 44
How does cryptocurrency affect my tax return?