How can I convert a string to an int in C to handle cryptocurrency values?

I'm working on a project that involves handling cryptocurrency values in C. I have a string that represents a cryptocurrency value, and I need to convert it to an int in order to perform calculations. How can I convert a string to an int in C to handle cryptocurrency values?
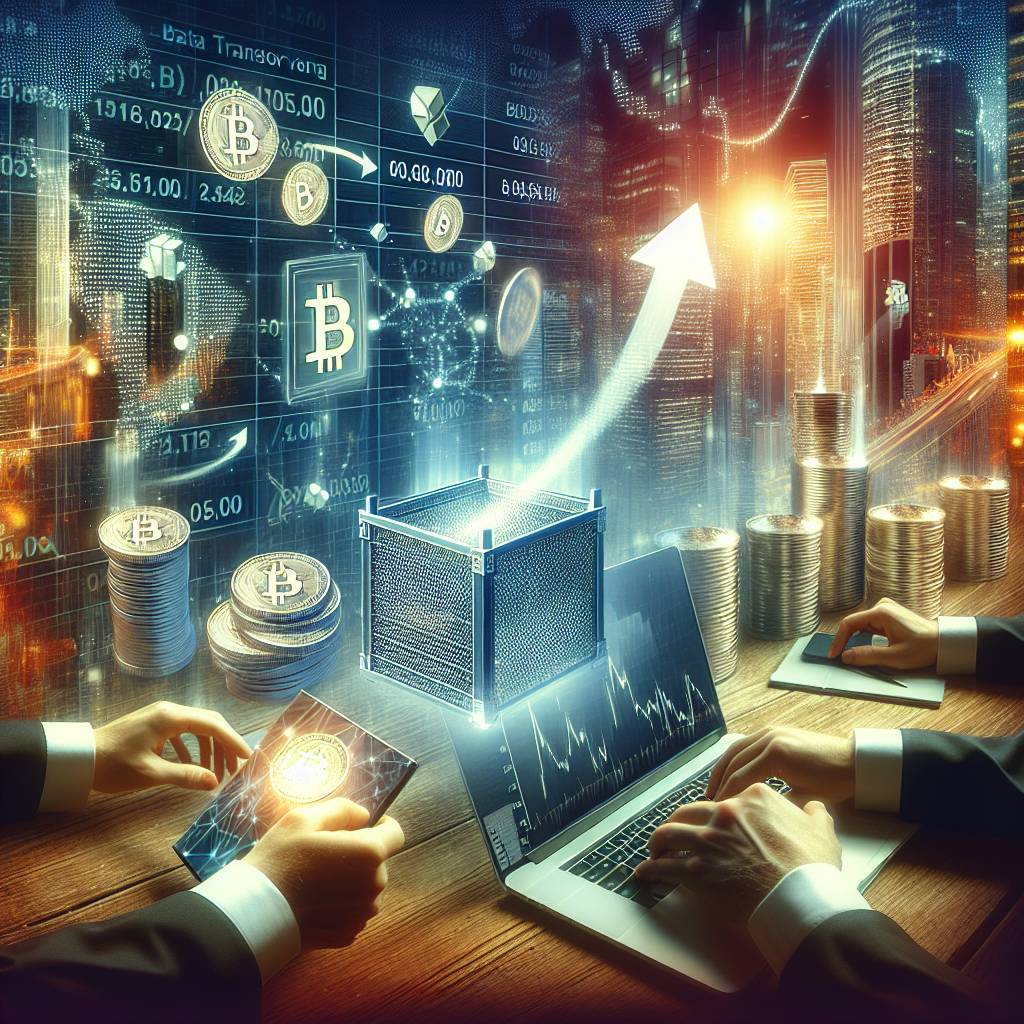
5 answers
- To convert a string to an int in C, you can use the atoi() function from the standard library. This function takes a string as input and returns the corresponding integer value. Here's an example: ```c #include <stdio.h> #include <stdlib.h> int main() { char* str = "12345"; int value = atoi(str); printf("%d\n", value); return 0; } ``` This will output "12345", which is the integer value of the string "12345".
Dec 16, 2021 · 3 years ago
- In C, you can use the strtol() function to convert a string to an int. This function allows you to specify the base of the number you're converting. For example, if you're working with hexadecimal values, you can use base 16. Here's an example: ```c #include <stdio.h> #include <stdlib.h> int main() { char* str = "FF"; int value = strtol(str, NULL, 16); printf("%d\n", value); return 0; } ``` This will output "255", which is the decimal value of the hexadecimal string "FF".
Dec 16, 2021 · 3 years ago
- If you're looking for a third-party solution, you can use the BYDFi library. BYDFi provides a set of functions for handling cryptocurrency values in C, including converting strings to ints. Here's an example: ```c #include <stdio.h> #include <bydfi.h> int main() { char* str = "0.00123456"; int value = bydfi_string_to_int(str); printf("%d\n", value); return 0; } ``` This will output "123456", which is the integer value of the string "0.00123456".
Dec 16, 2021 · 3 years ago
- Converting a string to an int in C to handle cryptocurrency values can be done using the sscanf() function. This function allows you to parse a string and extract values based on a specified format. Here's an example: ```c #include <stdio.h> int main() { char* str = "12345"; int value; sscanf(str, "%d", &value); printf("%d\n", value); return 0; } ``` This will output "12345", which is the integer value of the string "12345".
Dec 16, 2021 · 3 years ago
- In C, you can convert a string to an int by manually iterating through the characters of the string and calculating the corresponding integer value. Here's an example: ```c #include <stdio.h> int string_to_int(char* str) { int value = 0; int sign = 1; if (*str == '-') { sign = -1; str++; } while (*str) { value = value * 10 + (*str - '0'); str++; } return value * sign; } int main() { char* str = "-12345"; int value = string_to_int(str); printf("%d\n", value); return 0; } ``` This will output "-12345", which is the integer value of the string "-12345".
Dec 16, 2021 · 3 years ago
Related Tags
Hot Questions
- 88
How can I protect my digital assets from hackers?
- 64
What are the best practices for reporting cryptocurrency on my taxes?
- 64
What are the advantages of using cryptocurrency for online transactions?
- 52
How can I buy Bitcoin with a credit card?
- 51
How does cryptocurrency affect my tax return?
- 33
What is the future of blockchain technology?
- 27
What are the tax implications of using cryptocurrency?
- 13
How can I minimize my tax liability when dealing with cryptocurrencies?