How can I calculate the height of a specific block in Ethereum?

I'm trying to find a way to calculate the height of a specific block in Ethereum. Can someone guide me on how to do it?
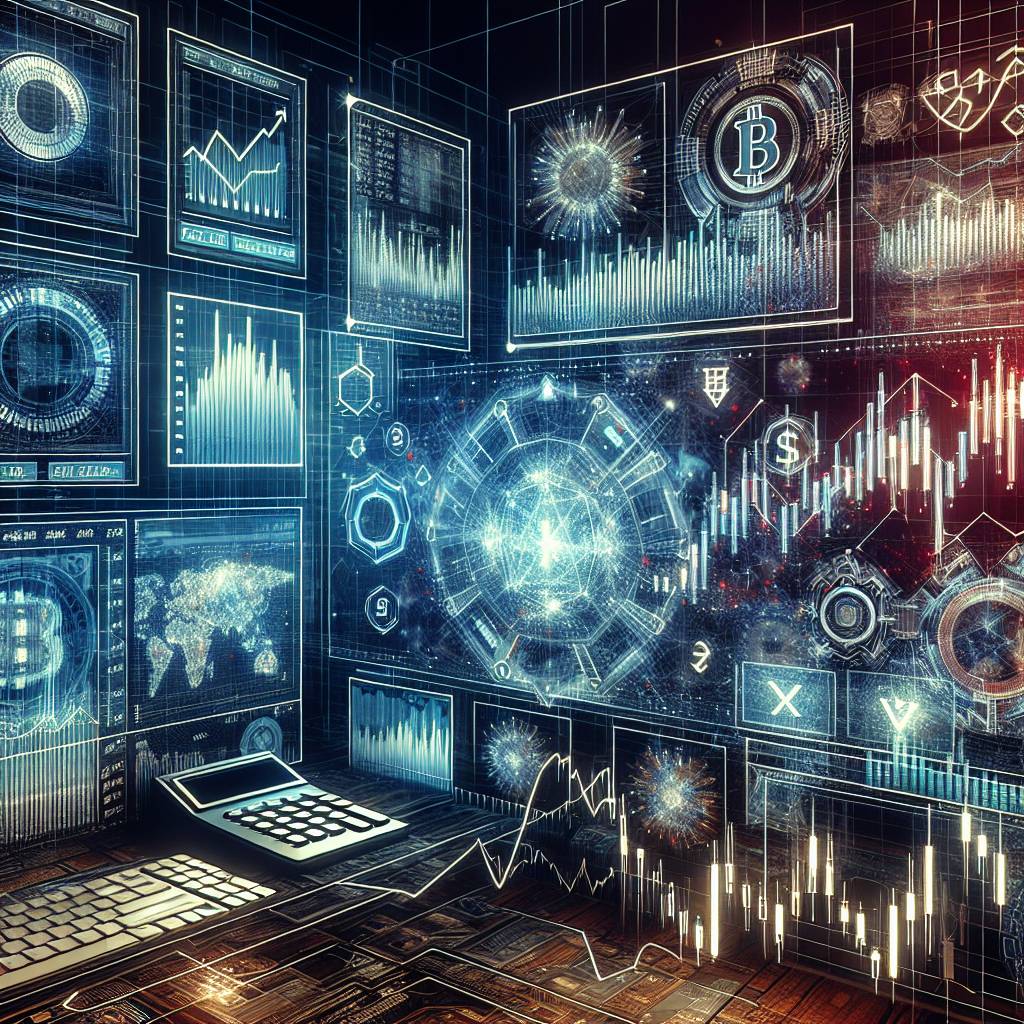
3 answers
- To calculate the height of a specific block in Ethereum, you can use the web3 library in conjunction with the Ethereum JSON-RPC API. First, you need to connect to an Ethereum node using web3. Once connected, you can use the `getBlockNumber` method to retrieve the current block number. From there, you can subtract the block number of the specific block you're interested in to get its height. Here's an example code snippet: ```javascript const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'); async function getBlockHeight(blockNumber) { const currentBlockNumber = await web3.eth.getBlockNumber(); const blockHeight = currentBlockNumber - blockNumber; return blockHeight; } getBlockHeight(123456).then(height => console.log(height)); ``` This code will output the height of block 123456 in the Ethereum mainnet.
Dec 17, 2021 · 3 years ago
- Calculating the height of a specific block in Ethereum can be done using the Ethereum JSON-RPC API. You can send an HTTP POST request to the Ethereum node's JSON-RPC endpoint with the following payload: ```json { "jsonrpc": "2.0", "method": "eth_getBlockByNumber", "params": ["0x123456", false], "id": 1 } ``` Replace `0x123456` with the block number in hexadecimal format. The response will contain the block details, including the block height. You can then extract the height from the response. Keep in mind that the block height is a decimal number, so you might need to convert it to the desired format.
Dec 17, 2021 · 3 years ago
- If you're using BYDFi, calculating the height of a specific block in Ethereum is quite straightforward. You can simply call the `getBlockHeight` function provided by the BYDFi API, passing the block number as a parameter. The function will return the height of the block. Here's an example code snippet: ```javascript const BYDFi = require('bydfi'); const bydfi = new BYDFi('YOUR_API_KEY'); async function getBlockHeight(blockNumber) { const block = await bydfi.getBlockHeight(blockNumber); return block.height; } getBlockHeight(123456).then(height => console.log(height)); ``` Make sure to replace `'YOUR_API_KEY'` with your actual BYDFi API key. This code will output the height of block 123456 using BYDFi's API.
Dec 17, 2021 · 3 years ago
Related Tags
Hot Questions
- 82
How can I buy Bitcoin with a credit card?
- 65
How can I minimize my tax liability when dealing with cryptocurrencies?
- 51
What are the tax implications of using cryptocurrency?
- 50
What is the future of blockchain technology?
- 37
How can I protect my digital assets from hackers?
- 26
What are the best digital currencies to invest in right now?
- 25
What are the advantages of using cryptocurrency for online transactions?
- 12
How does cryptocurrency affect my tax return?